Special Functions
This chapter describes the special functions provided by the PLT Scheme Science Collection.
The functions described in this chapter are defined in the special-functions sub-collection of the science collection. The entire special-functions sub-collection can be made available using the form:
(require (planet "special-functions.ss" ("williams" "science.plt")))
The individual modules in the special-functions sub-collection can also be made available as described in the sections below.
5.1 Error Functions
The error function is described in Abramowitz and Stegun, Chapter 7. The functions are defined in the error.ss
file in the special-functions sub-collection of the science collection and are made available using the form:
(require (planet "error.ss" ("williams" "science.plt") "special-functions"))
5.1.1 Error Function
Function:
(erf x) |
Contract: (-> real? (real-in -1.0 1.0)) |
|
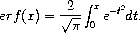
Example: Plot of (erf x) on the interval [ - 4, 4].
(require (planet "error.ss" ("williams" "science.plt") "special-functions")) (require (lib "plot.ss" "plot")) (plot (line erf) (x-min -4) (x-max 4) (y-min -1) (y-max 1) (title "Error Function, erf(x)"))
Figure 2 shows the resulting plot.
|
5.1.2 Complementary Error Function
Function:
(erfc x) |
Contract: (-> real? (real-in 0.0 2.0)) |
|

Example: Plot of (erfc x) on the interval [ - 4, 4].
(require (planet "error.ss" ("williams" "science.plt") "special-functions")) (require (lib "plot.ss" "plot")) (plot (line erfc) (x-min -4) (x-max 4) (y-min 0) (y-max 2) (title "Complementary Error Function, erfc(x)"))
Figure 3 shows the resulting plot.
|
5.1.3 Hazard Function
The hazard function for the normal distribution, also known as the inverse Mill's ratio, is the ratio of the probability function, P(x), to the survival function, S(x), and is defined as:
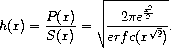
It decreases rapidly as x approaches - infty and asymptopes to h(x) x as x approaches + infty.
Function:
(hazard x) |
Contract: (-> real? (>=/c 0.0)) |
|
Example: Plot of (hazard x) on the interval [ - 5, 10].
(require (planet "error.ss" ("williams" "science.plt") "special-functions")) (require (lib "plot.ss" "plot")) (plot (line hazard) (x-min -5) (x-max 10) (y-min 0) (y-max 10) (title "Hazard Function, hazard(x)"))
Figure 4 shows the resulting plot.
|
5.2 Exponential Integral Functions
Information on the exponential integrals can be found in Abramowitz and Stegun, Chapter 5. The functions are defined in the exponential-integral.ss file in the special-functions sub-collection of the science collection and are made available using the form:
(require (planet "exponential-integral.ss" ("williams" "science.plt") "special-functions"))
5.2.1 Exponential Integral
Function:
(expint-E1 x) |
Function: (expint-E1-scaled x) |
Contract: (-> real? real?) |
|
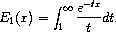
Example: Plot of (expint-E1 x) on the interval [ - 4, 4].
(require (planet "error.ss" ("williams" "science.plt") "special-functions")) (require (lib "plot.ss" "plot")) (plot (line expint_E1) (x-min -4) (x-max 4) (y-min -10) (y-max 10) (title "Exponential Integral E_1"))
Figure 5 shows the resulting plot.
|
Function:
(expint-E2 x) |
Function: (expint-E2-scaled x) |
Contract: (-> real? real?) |
|
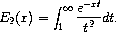
Example: Plot of (expint-E2 x) on the interval [ - 4, 4].
(require (planet "error.ss" ("williams" "science.plt") "special-functions")) (require (lib "plot.ss" "plot")) (plot (line expint_E2) (x-min -4) (x-max 4) (y-min -10) (y-max 10) (title "Exponential Integral E_2"))
Figure 6 shows the resulting plot.
|
5.2.2 Ei(x)
Function:
(expint-Ei-scaled x) |
Function: (expint-Ei x) |
Contract: (-> real? real?) |
|
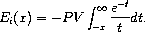
Example: Plot of (expint-Ei x) on the interval [ - 4, 4].
(require (planet "error.ss" ("williams" "science.plt") "special-functions")) (require (lib "plot.ss" "plot")) (plot (line expint_Ei) (x-min -4) (x-max 4) (y-min -10) (y-max 10) (title "Exponential Integral E_1"))
Figure 7 shows the resulting plot.
|
5.3 Gamma and Beta Functions
The gamma functions are defined in the gamma.ss file in the special-functions sub-collection of the science collection and are made available using the form:
(require (planet "gamma.ss" ("williams" "science.plt") "special-functions"))
Note that the gamma functions (Section 5.2), psi functions (Section 5.3), and the zeta functions (Section 5.4) are defined in the same module, gamma.ss. This is because their definitions are interdependent and PLT Scheme does not allow circular module dependencies.
5.3.1 Gamma Function
The gamma function is defined by the integral:

It is related to the factorial function by Gamma(n) = (n - 1)! for positive integer n. Further information on the gamma function can be found in Abramowitz & Stegun, Chapter 6.
Function:
(gamma x) |
Contract: (-> real? real?) |
|
Example: Plot of (gamma x) on the interval (0, 6].
(require (planet "gamma.ss" ("williams" "science.plt") "special-functions")) (require (lib "plot.ss" "plot")) (plot (line gamma) (x-min 0.001) (x-max 6) (y-min 0) (y-max 120) (title "Gamma Function, gamma(x)"))
Figure 8 shows the resulting plot.
|
Example: Plot of (gamma x) on the interval ( - 1, 0).
(require (planet "gamma.ss" ("williams" "science.plt") "special-functions")) (require (lib "plot.ss" "plot")) (plot (line gamma) (x-min -0.999) (x-max -0.001) (y-min -120) (y-max 0) (title "Gamma Function, gamma(x)"))
Figure 9 shows the resulting plot.
|
Function:
(lngamma x) |
Contract: (-> real? real?) |
|
Function:
(lngamma-sgn x) |
Contract: (-> real? (values real? (integer-in -1 1)) |
|
Example: Plot of (lngamma x) on the interval (0, 6].
(require (planet "gamma.ss" ("williams" "science.plt") "special-functions")) (require (lib "plot.ss" "plot")) (plot (line lngamma) (x-min 0.001) (x-max 6) (y-min 0) (y-max 120) (title "Log Gamma Function, lngamma(x)"))
Figure 10 shows the resulting plot.
|
Function:
(gamma-inv x) |
Contract: (-> real? real?) |
|
5.3.2 Regulated Gamma Function
The regulated gamma function is given by
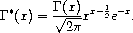
Function:
(gammastar x) |
Contract: (-> (>/c 0.0) real?) |
|
Example: Plot of (erf x) on the interval (0, 4].
(require (planet "gamma.ss" ("williams" "science.plt") "special-functions")) (require (lib "plot.ss" "plot")) (plot (line gammastar) (x-min 0.001) (x-max 4) (y-min 0) (y-max 10) (title "Regulated Gamma Function, gamma*(x)"))
Figure 11 shows the resulting plot.
|
5.3.3 Incomplete Gamma Function
Function:
(gamma-inc-Q a x) |
Contract: (-> (>/c 0.0) (>=/c 0.0) real?) |
|

for a > 0 and x > = 0.
Function:
(gamma-inc-Q a x) |
Contract: (-> (>/c 0.0) (>=/c 0.0) real?) |
|

for a > 0 and x > = 0.
Function:
(gamma-inc a x) |
Contract: (-> real? (>=/c 0.0) real?) |
|

for a real and x > = 0.
5.3.4 Factorial Function
The factorial of a positive integer n, n!, is defined as n! = n × (n - 1) × ... × 2 × 1. By definition, 0! = 1. The factorial function is related to the gamma function by n! = Gamma(n + 1).
Function:
(fact n) |
Contract: (-> natural-number? (>/c 1.0)) |
|
Function:
(lnfact n) |
Contract: (-> natural-number? (>=/c 0.0)) |
|
5.3.5 Double Factorial Function
The double factorial of n, n!!, is defined as n!! = n × (n - 2) × (n - 4) × .... By definition, - 1!! = 0!! = 1.
Function:
(double-fact n) |
Contract: (-> natural-number? (>/c 1.0)) |
|
Function:
(lndouble-fact n) |
Contract: (-> natural-number? (>=/c 0.0)) |
|
5.3.6 Binomial Coefficient Function
The binomial coefficient, b choose m, is defined as:
![[specialfunctions-Z-G-22.gif]](specialfunctions-Z-G-22.gif)
Function:
(choose n m) |
Contract: (-> natural-number? natural-number? (>/c 1.0)) |
|
Function:
(lnchoose n m) |
Contract: (-> natural-number? natural-number? (>/c 0.0)) |
|
log (n choose m).
5.3.7 Beta Functions
Function:
(beta a b) |
Contract: (-> (>/c 0.0) (>/c 0.0) real?) |
|

for a > 0 and b > 0.
Function:
(beta a b) |
Contract: (-> (>/c 0.0) (>/c 0.0) real?) |
|
5.4 Psi Functions
The psi functions are defined in gamma.ss in the special-functions sub-collection of the science collection and are made available using the form:
(require (planet "gamma.ss" ("williams" "science.plt") "special-functions"))
Note that the gamma functions (Section 5.2), psi functions (Section 5.3), and zeta functions (Section 5.4) are defined in the same module, gamma.ss. This is because their definitions are interdependent and PLT Scheme does not allow circular module dependencies.
5.4.1 Psi (Digamma) Functions
Function:
(psi-int n) |
Contract: (-> (integer-in 1 +inf.0) real?) |
|
Function:
(psi x) |
Contract: (-> real? real?) |
|
Function:
(psi-1piy y) |
Contract: (-> real? real?) |
|
Example: Plot of (psi x) on the interval (0, 5].
(require (planet "gamma.ss" ("williams" "science.plt") "special-functions")) (require (lib "plot.ss" "plot")) (plot (line psi) (x-min 0.001) (x-max 5) (y-min -5) (y-max 5) (title "Psi (Digamma) Function, psi(x)"))
Figure 12 shows the resulting plot.
|
5.4.2 Psi-1 (Trigamma) Functions
Function:
(psi-1-int n) |
Contract: (-> (integer-in 1 +inf.0) real?) |
|
Function:
(psi-1 x) |
Contract: (-> real? real?) |
|
Example: Plot of (psi-1 x) on the interval (0, 5].
(require (planet "gamma.ss" ("williams" "science.plt") "special-functions")) (require (lib "plot.ss" "plot")) (plot (line psi-1) (x-min 0.005) (x-max 5) (y-min 0) (y-max 5) (title "Psi-1 (Trigamma) Function, psi-1(x)"))
Figure 13 shows the resulting plot.
|
5.4.3 Psi-n (Polygamma) Function
Function:
(psi-n n x) |
Contract: (-> natural-number? (>/c 0.0) real?) |
|
Example: Plot of (psi-n n x) on the interval (0, 5].
(require (planet "gamma.ss" ("williams" "science.plt") "special-functions")) (require (lib "plot.ss" "plot")) (plot (line (lambda (x) (psi-n 3 x))) (x-min 0.05) (x-max 5) (y-min 0) (y-max 10) (title "Psi-n (Polygamma) Function, psi-n(3, x)"))
Figure 14 shows the resulting plot.
|
5.5 Zeta Functions
The Riemann zeta function is defined in Abramowitz and Stegun, Section 23.2. The zeta functions are defined in the gamma.ss file in the special-functions sub-collection of the science collection and are made available using the form:
(require (lib "gamma.ss" ("williams" "science.plt") "special-functions"))
Note that the gamma functions (Section 5.2), psi functions (Section 5.3), and zeta functions (Section 5.4) are defined in the same module, gamma.ss. This is because their definitions are interdependent and PLT Scheme does not allow circular module dependencies.
5.5.1 Riemann Zeta Functions
The Riemann zeta function is defined by the infinite sum

Function:
(zeta-int n) |
Contract: (-> integer? real?) |
|
Function:
(zeta s) |
Contract: (-> real? real?) |
|
Example: Plot of (zeta x) on the interval [ - 5, 5].
(require (planet "gamma.ss" ("williams" "science.plt") "special-functions")) (require (lib "plot.ss" "plot")) (plot (line zeta) (x-min -5) (x-max 5) (y-min -5) (y-max 5) (title "Reimann Zeta Function, zeta(x)"))
Figure 15 shows the resulting plot.
|
5.5.2 Riemann Zeta Functions Minus One
For large positive arguments, the Reimann zeta function approaches one. In this region the fractional part is interesting amd, therefore, we need a function to evaluate it explicitly.
Function:
(zetam1-int n) |
Contract: (-> integer? real?) |
|
Function:
(zetam1 s) |
Contract: (-> real? real?) |
|
5.5.3 Hurwitz Zeta Function
The Hurwitx zeta function is defined by
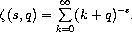
Function:
(hzeta s q) |
Contract: (-> (>/c 1.0) (>/c 0.0) real?) |
|
Example: Plot of (hzeta x) on the interval (1, 5].
(require (planet "gamma.ss" ("williams" "science.plt") "special-functions")) (require (lib "plot.ss" "plot")) (plot (line (lambda (x) (hzeta x 2.0))) (x-min 1.001) (x-max 5) (y-min 0) (y-max 5) (title "Hurwitz Zeta Function, hzeta(x, 2.0)"))
Figure 16 shows the resulting plot.
|
5.5.4 Eta Functions
The eta function is defined by

Function:
(eta-int n) |
Contract: (-> integer? real?) |
|
Function:
(eta s) |
Contract: (-> real? real?) |
|
Example: Plot of (eta x) on the interval [ - 10, 10].
(require (planet "gamma.ss" ("williams" "science.plt") "special-functions")) (require (lib "plot.ss" "plot")) (plot (line eta) (x-min -10) (x-max 10) (y-min -5) (y-max 5) (title "Eta Function, eta(x)"))
Figure 17 shows the resulting plot.
|